Rock Paper Scissors Game in Python:
We can use the PyCharm code editor for this example. If you do not know about it then follow this link- How to install PyCharm for Python and create a program in it. |
Rock Paper Scissors Game Rules:
- Rock smashes Scissors- Rock wins.
- Paper covers Rock- Paper wins.
- Scissors cuts Paper- Scissors wins.
Code Unit:
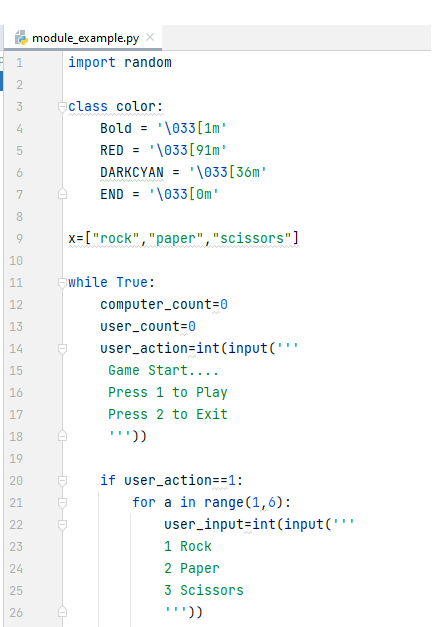
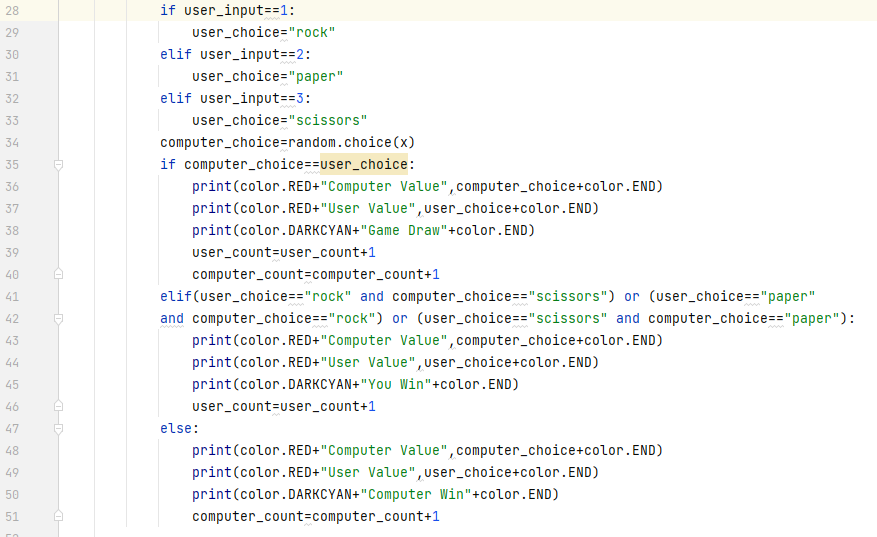
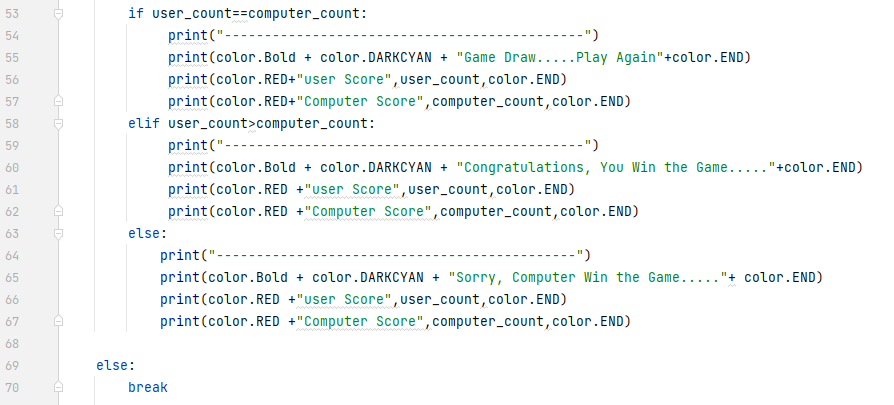
Output:
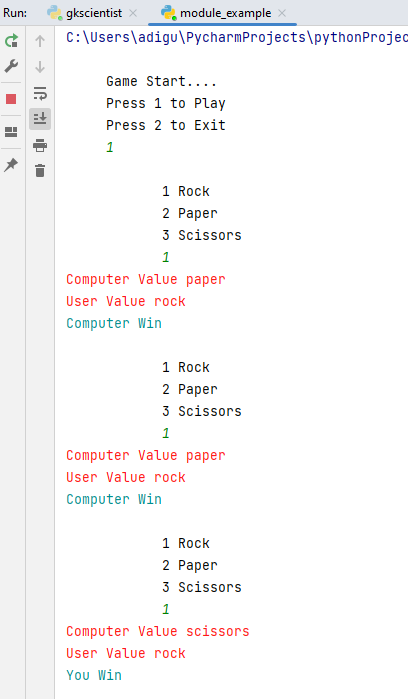
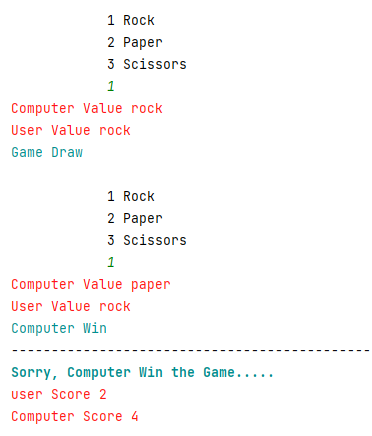
Rock Paper Scissors Game in Python Source Code: Do proper Code Indentation otherwise, the Source Code does not work and gives an error.
import random
class color:
Bold = '\033[1m'
RED = '\033[91m'
DARKCYAN = '\033[36m'
END = '\033[0m'
x=["rock","paper","scissors"]
while True:
computer_count=0
user_count=0
user_action=int(input('''
Game Start...
Press 1 to Play
Press 2 to Exit
'''))
if user_action==1:
for a in range(1,6):
user_input=int(input('''
1 Rock
2 Paper
3 Scissors
'''))
if user_input == 1:
user_choice ="rock"
elif user_input == 2:
user_choice ="paper"
elif user_input == 3:
user_choice ="scissors"
computer_choice = random.choice(x)
if computer_choice == user_choice:
print(color.RED +"Computer Value", computer_choice + color.END)
print(color.RED +"User Value", user_choice + color.END)
print(color.DARKCYAN +"Game Draw"+color.END)
user_count = user_count + 1
computer_count = computer_count + 1
elif(user_choice =="rock" and computer_choice == "scissors") or (user_choice =="paper"
and computer_choice == "rock") or (user_choice =="scissors" and computer_choice == "paper"):
print(color.RED +"Computer Value", computer_choice + color.END)
print(color.RED +"User Value", user_choice + color.END)
print(color.DARKCYAN +"You Win"+color.END)
user_count = user_count + 1
else:
print(color.RED +"Computer Value", computer_choice + color.END)
print(color.RED +"User Value", user_choice + color.END)
print(color.DARKCYAN +"Computer Win"+color.END)
computer_count = computer_count + 1
if user_count == computer_count:
print("-----------------------------------------")
print(color.Bold + color.DARKCYAN + "Game Draw.....Play Again"+color.END)
print(color.RED +"user Score", user_count, color.END)
print(color.RED +"Computer Score", computer_count, color.END)
elif user_count > computer_count:
print("-----------------------------------------")
print(color.Bold + color.DARKCYAN + "Congratulations, You Win the Game......."+color.END)
print(color.RED +"user Score", user_count, color.END)
print(color.RED +"Computer Score", computer_count, color.END)
else:
print("-----------------------------------------")
print(color.Bold + color.DARKCYAN + "Sorry, Computer Win the Game......."+ color.END)
print(color.RED +"user Score", user_count, color.END)
print(color.RED +"Computer Score", computer_count, color.END)
else:
break
Comments (No)