Create Java FileChooser:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
Class JFileChooser displays a dialog that enables the user to easily select files or directories.
Java FileChooser Constructors:
Constructor | Description |
---|---|
JFileChooser( ) | This form of constructor creates a JFileChooser pointing to the user’s default directory. |
JFileChooser(String path) | This form of constructor creates a JFileChooser using the given path. |
JFileChooser(File f) | This form of constructor creates a JFileChooser using the given file as the path. |
Java FileChooser Methods:
Method | Description |
---|---|
int showOpenDialog(Component parent) | Pops up an “Open File” FileChooser dialog. |
int showSaveDialog(Component parent) | Pops up a “Save File” FileChooser dialog. |
File getCurrentDirectory( ) | Returns the current directory. |
File getSelectedFile( ) | Returns the selected file. |
void setCurrentDiretory(File dir) | Sets the current directory. |
Sample Code to Create FileChooser in Java:
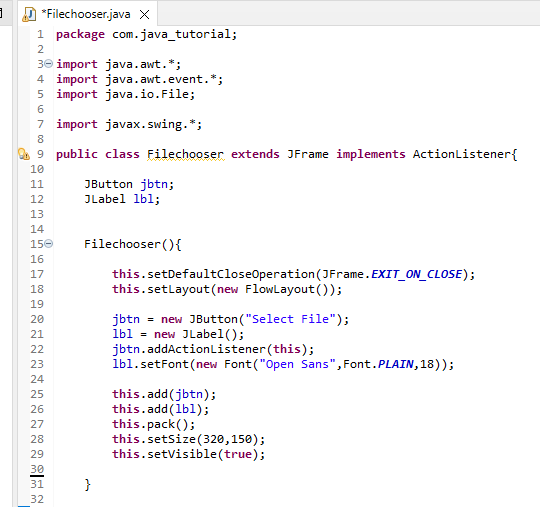
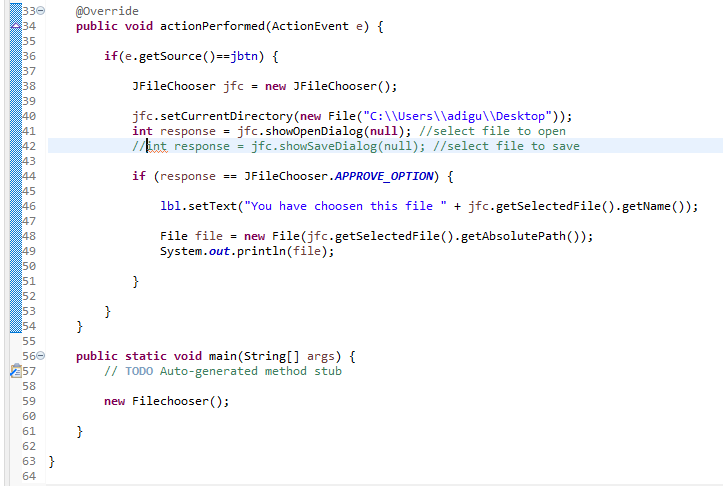
Output:
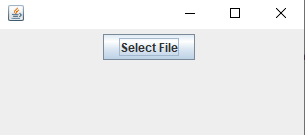
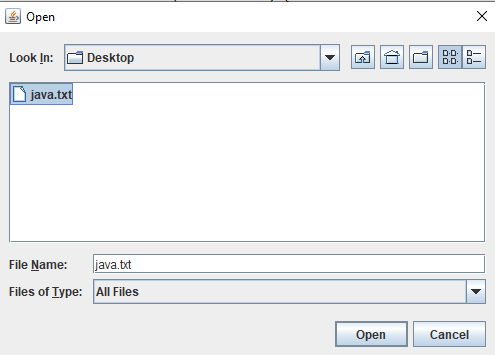
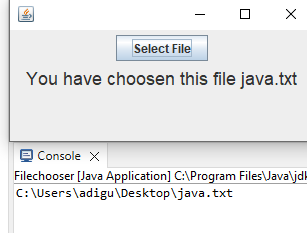
import java.awt.*;
import java.awt.event.*;
import java.io.File;
import javax.swing.*;
public class Filechooser extends JFrame implements ActionListener{
JButton jbtn;
JLabel lbl;
Filechooser(){
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setLayout(new FlowLayout());
jbtn = new JButton("Select File");
lbl = new JLabel();
jbtn.addActionListener(this);
lbl.setFont(new Font("Open Sans",Font.PLAIN,18));
this.add(jbtn);
this.add(lbl);
this.pack();
this.setSize(320,150);
this.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource()==jbtn) {
JFileChooser jfc = new JFileChooser();
jfc.setCurrentDirectory(new File("C:\\Users\\adigu\\Desktop"));
int response = jfc.showOpenDialog(null); //select file to open
//int response = jfc.showSaveDialog(null); //select file to save
if (response == JFileChooser.APPROVE_OPTION) {
lbl.setText("You have choosen this file " + jfc.getSelectedFile().getName());
File file = new File(jfc.getSelectedFile().getAbsolutePath());
System.out.println(file);
}
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
new Filechooser();
}
}
Comments (No)