Java Wrapper Classes:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
You can treat primitive types as objects by using Java’s wrapper classes. A wrapper class holds a primitive type and provides methods to perform common functions related to that primitive type. The following table shows the classes defined by Java corresponding to different primitive types:
Primitive Type | Wrapper Class |
---|---|
byte | java.lang.Byte |
short | java.lang.Short |
int | java.lang.Integer |
long | java.lang.Long |
float | java.lang.Float |
double | java.lang.Double |
char | java.lang.Character |
boolean | java.lang.Boolean |
void | java.lang.Void |
Autoboxing and Unboxing of Primitives: In Java, the compiler automatically wraps primitives in their wrapper types and unwraps them where appropriate. This process is called autoboxing and unboxing the primitive. It happens when primitives are used as arguments and return values in methods and on simple assignment to variables.
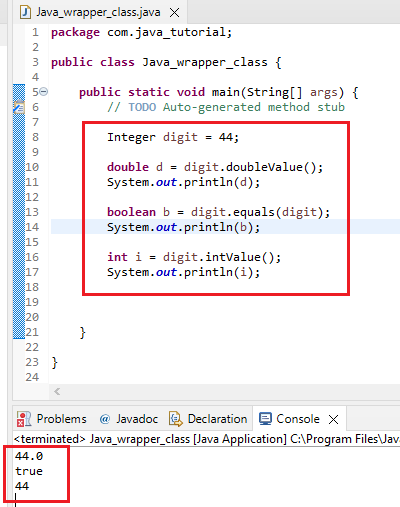
public class Java_wrapper_class {
public static void main(String[] args) {
// TODO Auto-generated method stub
Integer digit = 44;
double d = digit.doubleValue();
System.out.println(d);
boolean b = digit.equals(digit);
System.out.println(b);
int i = digit.intValue();
System.out.println(i);
}
}
Comments (No)