Table of Contents
Frequently Asked Java Programming Questions in Interview:
Ques. What is a Java programming language?
Ans. Java is an object-oriented, high-level interpreted programming language and it also provides us with features such as security, robustness, multithreading, and platform independence. In 1991, James Gosling developed the Java programming language.
Ques. List out all the features of the Java programming language.
Ans. Java provides the following features:
- Architecture Neutral
- Interpreted
- Dynamic
- Distributed
- Multithreaded
- High Performance
- Robust
- Secured
- Platform Independent
- Portable
- Simple
- Object-Oriented
Ques. Why is Java programming language considered a platform-independent programming language?
Ans. Java is a platform-independent programming language and programs written in Java language do not depend on software and hardware as its code is compiled by a compiler and then the code is converted into byte code which is platform-independent and can run on any machine on which JRE(Java Runtime Environment) is installed.
Ques. Java is a pure object-oriented language or not. Support your answer with a reason.
Ans. Java is not a pure object-oriented language as primitive data types such as double, long, float, int, short, char, Boolean, and byte are supported by it.
Ques. What is the difference between Java and C++?
Ans. The difference between Java and C++ programming language is explained below:
- Java is both interpreted and compiled language whereas C++ is compiled language.
- Java is a platform-independent programming language whereas programs written in C++ can only run on the machine on which it is compiled.
- Pointers are allowed to be used by users in C++ whereas java does not allow users to use pointers.
- Multiple inheritances are supported in the C++ programming language whereas Java does not support multiple inheritances.
Ques. Why are pointers not supported in the Java programming language?
Ans. For programming beginners, pointers are considered somewhat complicated and unsafe. And code simplicity is the main focus of the Java programming language. And pointers usage can become a challenge for programmers. And apart from that by the use of pointers security is also compromised as the pointers allow memory access directly.
And the garbage collection process also becomes slow by the usage of pointers. So references are used by Java in place of pointers. As references can not be modified like the pointers.
Ques. Explain the difference between JVM, JRE, and JDK
Ans. JVM: JVM stands for Java Virtual Machine. And JVM is a virtual machine by which a runtime environment is provided for the execution of the byte code. Oracle and other companies have provided its implementation.
JRE: Java Runtime Environment is abbreviated as JRE. And JRE is an implementation of JVM. The software toolset for Java application development is known as the JRE. Runtime Environment is provided by the JRE. A set of libraries and other files is included in it.
JDK: JDK stands for Java Development Kit. JDK is a software development environment and it is used for Java applications and applet development. JRE and development tools are included in it.
Ques. Tell me something about the JIT compiler.
Ans. JIT compiler stands for Just In Time compiler. And this compiler is used for performance improvement by compiling similar functionality byte code simultaneously. Compilation time is reduced by the JIT compiler. And here compiler means conversion of the JVM instruction set into a particular CPU instruction set.
Ques. Why java is called ‘write once and run anywhere’?
Ans. Byte code of the Java programming language provides it with the feature of writing once and running anywhere. A program written in Java is converted into a class file or bytecode by the Java compiler. And bytecode is the intermediate code between source and machine code. And the bytecode is platform-independent and can be executed on any machine. So a Java program written on any machine can be executed anywhere because of its intermediate bytecode conversion.
Ques. Explain the concept of local and instance variables in Java.
Ans. Instance variables are those variables that are declared inside the class but outside of every method of the class. And it can be accessed by every method of the class. Object properties are described by the instance variables and these are bound for an object.
Every class object has its copy of instance variables. And in case of any manipulation of instance variables, only that particular object variable is impacted, all other object variables are not impacted.
Example of instance variable:
class Example{
public String name;
public int age;
public String address;
public int dob;
}
Local variables are those variables that can be declared inside the method, constructor or block and can only be accessed inside that particular block. If the local variables are declared inside the particular method then the other method of that class does not have any knowledge about those variables.
Example of Local variables:
public void example() {
String name;
int age;
String address;
int dob;
}
Ques. How can an infinite loop be declared in Java?
Ans. Infinite loops run for infinite iterations and do not have any breaking conditions. Infinite loop in Java can be declared in the following ways:
Infinite for loop
for(;;){
// Task needs to be executed
// infinite number of times
}
Infinite while loop
while(true){
// Task needs to be executed
// infinite number of times
}
Infinite do-while loop
do{
// Task needs to be executed
// infinite number of times
}while(true);
Ques. The main method can be overloaded in Java or not.
Ans. Yes, the main method overloading can also be achieved. We can create multiple overloaded main methods as per our requirements. And main with the definition public static void main(string[] args) is only called by the JVM
Ques. What do you understand by constructor overloading?
Ans. Constructor overloading is the way of creating multiple constructors in the class having the same name but a different number of arguments, sequence of arguments, or data type of parameters. Compilers recognize various constructors with the help of the number of arguments and data types of arguments passed through them.
Ques. What values are by default assigned to the instances and variables in Java?
Ans. In Java, by default, no value is assigned to the variables and before using the variable its initialization is required. And java will throw compilation errors if the initialization is not done.
- But if we talk about instances then the instance variables are automatically initialized with default values at the time of object creation by the default constructor based on the variable data type.
- Null is assigned in case of reference
- And 0 value is assigned to the numeric data type variables.
Ques. Explain the concept of data encapsulation in Java
Ans.
- Data encapsulation is one of the pillars of object-oriented programming which means wrapping data and behavior into a single unit.
- Data encapsulation is also used for the security of object private properties and hence it is also used to achieve the data hiding feature.
Ques. Explain the concept of this keyword in Java
Ans. This keyword in Java is a reference variable that is mainly used for referring to the object of the current class. There are many different usages of this keyword in Java. Current class properties like a constructor, variables, method, etc. can also be accessed using this keyword. This keyword can also be used as a parameter for passing in the methods and constructors. We can also use this keyword for returning the instance of the current class from the method.
Ques. Static methods can be overridden or not?
Ans. No static methods can not be overridden.
Ques. Why the main method is static in Java
Ans. As for calling the static method we do not need to create an object. And if the main method is made static then the JVM is required to create an object for calling the main method which will lead to extra memory allocation.
Ques. Explain the constructor concept in Java.
Ans. Constructor is a special type of method that explicitly does not return any value and has the name the same as the class name and it is used for object state initialization. Constructor invocation is done at the time of memory allocation to the object. Constructor is called whenever the object is created with the help of this keyword.
Ques. Explain types of constructors in Java.
Ans. There are two types of the constructor based on arguments passed to them:
Default constructor: Any argument is not passed in the default constructor and the main use of the default constructor is the default values initialization of instance variables. A default constructor is also used for doing tasks at the time of object creation. If no constructor is defined in the class then the compiler implicitly invokes the default constructor.
Parameterized constructor: Parameterized constructors are used for instance variables initialization with the provided values. And arguments are also accepted by the parameterized constructor.
Ques. Can constructors be overloaded in Java?
Ans. Yes, constructors are allowed to be overloaded in Java by modifying the number of arguments, data type, or sequence of arguments passed to the constructor.
Ques. Can the constructor be made final in Java?
Ans. No constructors are not allowed to be final in Java.
Ques. Does any value is returned by the constructor?
Ans. Constructor explicitly doesn’t return any value but the current class instance is returned by the constructor implicitly.
Ques. Explain the concept of an object.
Ans. An object is a real-world entity that has its state and behavior. An object is the class instance and the object has instance variables that will behave like the state of an object and the methods will behave like the object behavior. A new keyword is used in Java for object creation.
Ques. Discuss the advantages of using packages in Java.
Ans. Some of the advantages of using packages in Java are given below:
- Name clashes are avoided by the packages
- Access control becomes easier with the help of packages.
- Classes can be easily organized.
Ques. Explain about different access specifiers available in Java
Ans. Access specifiers used in Java are used for defining the scope of variables, classes, or methods. Four types of access specifiers are available in Java.
Public: Public variables, methods, and classes can be accessed anywhere by any method or class.
Protected: Those variables, methods, and classes defined with the protected keyword can be accessed by the same package classes and by sub-classes of other packages.
Default: If any access specifier is not specified then by default variables, methods, and classes are default and can be accessed within the same package.
Private: Those methods and variables which are declared with the private keyword can be accessed within that class.
Ques. What are static variables and methods and what is their purpose?
Ans. The static variables or methods are shared among all the objects of the class; it does not belong to a particular object of the class. And static variables and methods can be accessed without creating an object of the class. So static is used with variables or methods for defining methods and variables which are common to every object of the class.
Ques. Explain the difference between method overriding and method overloading.
Ans. Method overloading in Java can be achieved by defining the methods with the same name in the same class. But the methods have different parameter signatures such as parameters that can differ in their sequence, data types, and number of arguments. Program readability is increased by the method overloading and it can be achieved in a single class. Method overloading can not be achieved by different return types of methods.
Method overriding is achieved when two classes have the same methods with the same signature and the inheritance relationship is associated with the classes. The derived class can provide the specific implementation of the methods already defined in the base class using the method overriding.
Ques. Explain the inheritance concept in Java.
Ans. Inheritance is an object-oriented programming feature that allows the creation of new classes based on the features of an existing class. Inheritance is used to achieve code reusability and method overriding. When the existing class properties are inherited then we can reuse the parent class methods and variables. IS-A and the parent-child relationship are represented through inheritance.
Four types of inheritance are available in Java programming language:
- Single-level inheritance
- Multi-level inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
We can not achieve multiple inheritance in Java using classes.
Ques. Which is the superclass of all classes in Java
Ans. In Java, the object class is the superclass of all the classes.
Ques. Why is the super keyword used in Java?
Ans. The super keyword is a reference variable that is used to refer to the object of the immediate parent of the class and to access the immediate parent class constructor and method. Parent class instance is implicitly created during subclass instance creation and it is referred to by using the super keyword. And inside the sub-class constructor, super() is automatically added by the compiler if there is no this or super.
Ques. Can we achieve method overriding on private methods
Ans. No private methods can not be overridden.
Ques. Explain the concept of the final keyword.
Ans. The final keyword can be used with methods, variables, and class. If the variable is declared as final then it will behave like a constant and we can not change its value after initialization. And a method declared with the final keyword can not be overridden. And the final class can not be inherited.
Conclusion:
- Java is an interpreted, secure, platform-independent object-oriented programming.
- There are two types of constructors in Java i.e. default constructor and parameterized.
- Multiple inheritances is not supported in Java.
- Four types of access specifiers are available in Java i.e. public, private, protected, and default.
- Four types of inheritance are supported in Java.
Written By Ankit Dixit:
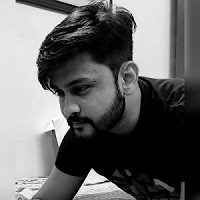
Ankit Dixit is a content specialist with 3+ years of experience and he is passionate about creating compelling and informative content that engages readers and delivers value in writing about cutting-edge technologies, including programming, cloud computing, web development, and cyber security.
Comments (No)