Inheritance in Java:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
Inheritance allows a class to gain the properties or methods of another class. The class which adheres the properties is known as derived class or subclass and the one which gives its properties is known as base class or super class. The derived class can add its own additional variables and methods. These additional variable and methods differentiated the derived class from the base class. The keyword used for inheritance is extends.
Why we use inheritance in Java?
- For method overriding (so runtime polymorphism can be achieved).
- For code reusability.
Types of Inheritance:
(1) Single Inheritance- When a single class is inherited from a single base class, it is called single inheritance.
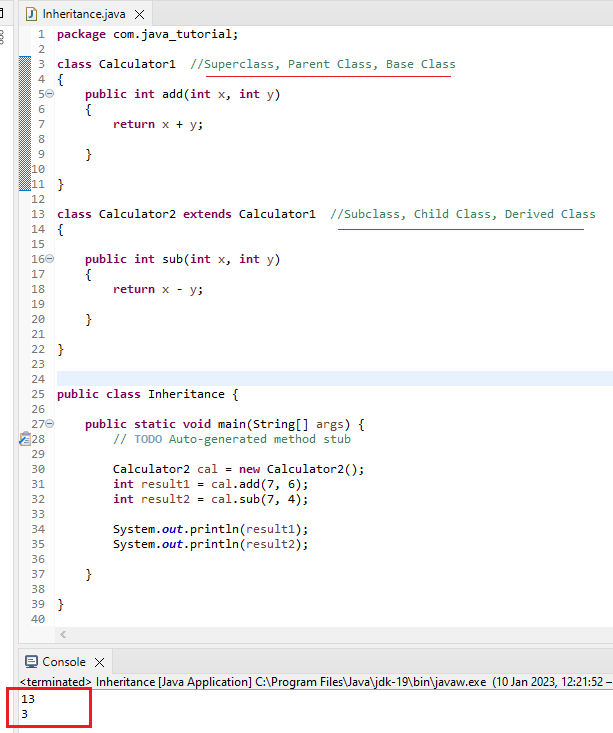
class Calculator1 //Superclass, Parent Class, Base Class
{
public int add(int x, int y)
{
return x + y;
}
}
class Calculator2 extends Calculator1 //Subclass, Child Class, Derived Class
{
public int sub(int x, int y)
{
return x - y;
}
}
public class Inheritance {
public static void main(String[] args) {
// TODO Auto-generated method stub
Calculator2 cal = new Calculator2();
int result1 = cal.add(7, 6);
int result2 = cal.sub(7, 4);
System.out.println(result1);
System.out.println(result2);
}
}
(2) Multilevel Inheritance- In multilevel inheritance, a subclass is used as a superclass for another class.
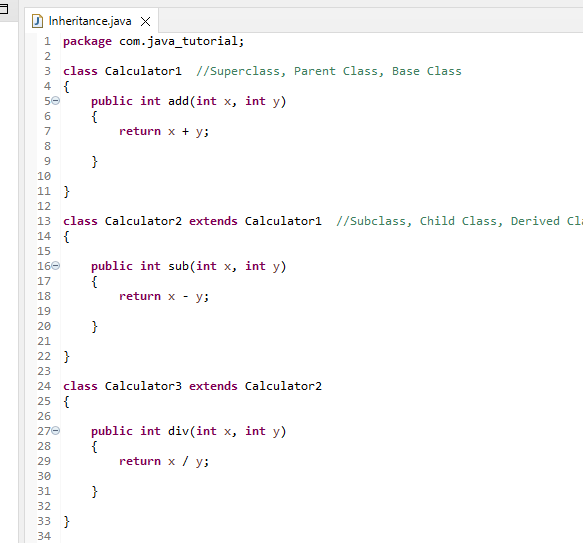
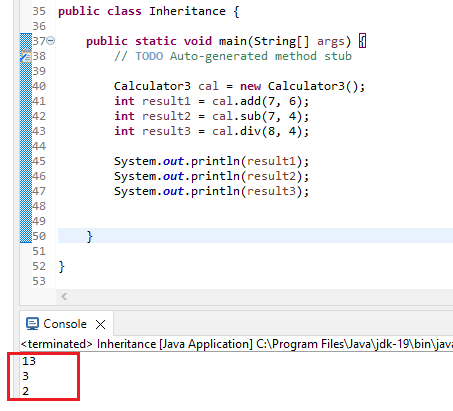
class Calculator1 //Superclass, Parent Class, Base Class
{
public int add(int x, int y)
{
return x + y;
}
}
class Calculator2 extends Calculator1 //Subclass, Child Class, Derived Class
{
public int sub(int x, int y)
{
return x - y;
}
}
class Calculator3 extends Calculator2
{
public int div(int x, int y)
{
return x / y;
}
}
public class Inheritance {
public static void main(String[] args) {
// TODO Auto-generated method stub
Calculator3 cal = new Calculator3();
int result1 = cal.add(7, 6);
int result2 = cal.sub(7, 4);
int result3 = cal.div(8, 4);
System.out.println(result1);
System.out.println(result2);
System.out.println(result3);
}
}
(3) Hierarchical Inheritance- When multiple subclasses inherit the characteristics from one superclass, then it is known as hierarchical inheritance.
(4) Multiple Inheritance- When one single class inherits the characteristics of multiple superclasses then it is known as multiple inheritance. For achieving multiple inheritance in Java, interfaces can be used.
Why Java does not support multiple inheritance? If multiple inheritance is used in Java, it can lead to ambiguity. If a method with the same name exists in multiple superclasses, then on calling that method the compiler will not be to determine as to which of the methods in the superclasses is to be called, leading to error or incorrect result.
(5) Hybrid Inheritance- When one or more types of inheritance are combined together, it is known as hybrid inheritance. If one of the combinations in a hybrid inheritance is multiple inheritance then that hybrid inheritance is not supported by Java. For example- if you combine hierarchical and multilevel inheritance together then this becomes hybrid inheritance and Java supports it. But if you combine multiple inheritance with some other type of inheritance then it is not supported by Java.
Comments (No)