Queue in Python:
We can use the PyCharm code editor for this example. If you do not know about it then follow this link- How to install PyCharm for Python and create a program in it. |
(1) Queue is an ordered linear list of elements, having different ends for adding and removing elements in it. It works on First-In-First-Out (FIFO) principle, meaning an element that comes in first goes out first when a value is read from a queue. A queue has two main operations called enqueue and dequeue. Enqueue is used to insert a new element to the queue at the rear end. Dequeue is used to remove one element at a time from the front of the queue.
(2) Example of Implementation of Queue in Python:
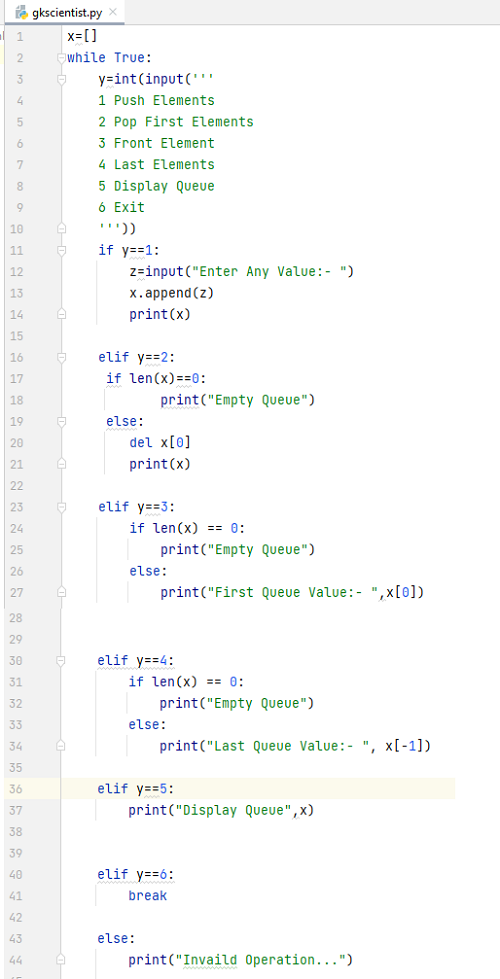
Now run the code, and you got the following output as shown below.
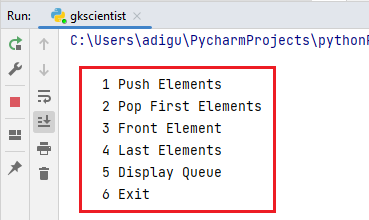
Now press 1 in the output window, in order to use the Push operation. After pressing 1, you got the message “enter any value”. You add any value of your choice. If you want to add another value then again click on 1 and you got the same message “enter any value”. You can add as many values as you want. The output is shown below.
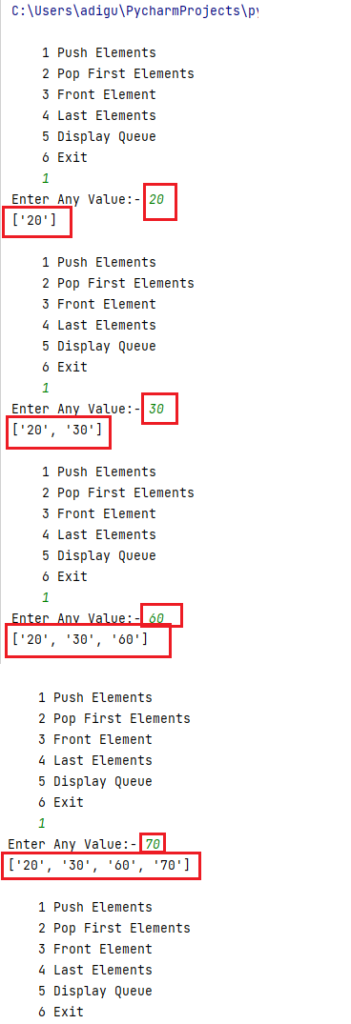
Now the list is created by using the push operation. Now press 2 in the output window, in order to use the Pop operation. After pressing 2, ‘20’ will be dropped from the list as shown below.
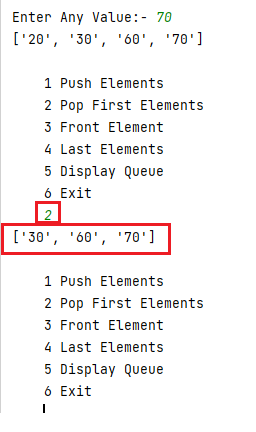
After pressing 3 we get the first element of the list as shown below.
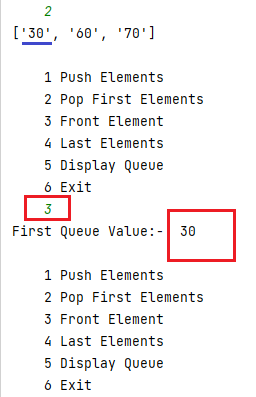
After pressing 4 we get the last element of the list as shown below.
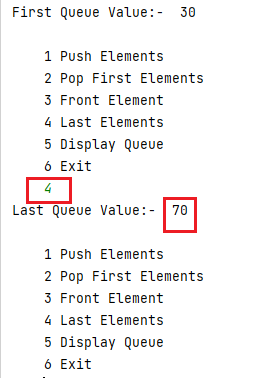
Now press 5 in the output window, in order to use the Display operation. This operation displays the full queue as shown below. You got only three values in the list because you have dropped the first value already by using the pop operation.
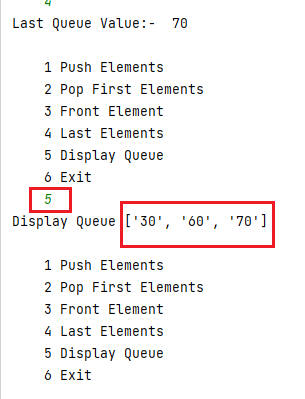
If you press 6, the process is getting finished.
Note: In the above example, we have performed a step-by-step operation. If you press 2 before 1, then the pop operation comes into play and you got the message “Empty Queue” as we set in the code as shown above because you did not create the list, so there will be no data that is why you got the output “Empty Queue”. The same is apply to all conditions.
Comments (No)