Java Switch Statement:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
Java switch statement provides an alternative to complicated multiple if else statements. It compares the value of a variable/expression against a set of predefined values. The statements within the exact match are executed. It supports numbers, String, enumerations as well as objects of Wrapper classes such as Character, Byte, Short, and Integer as variable/expression for a switch-case. This statement is created using the switch keyword followed by a variable/expression in parentheses ( ). Based on the value of the expression, the appropriate case is executed which is indicated by the case keyword. Each case is terminated by the break keyword. If none of the cases match the expression, the default case is executed.
Example 1:
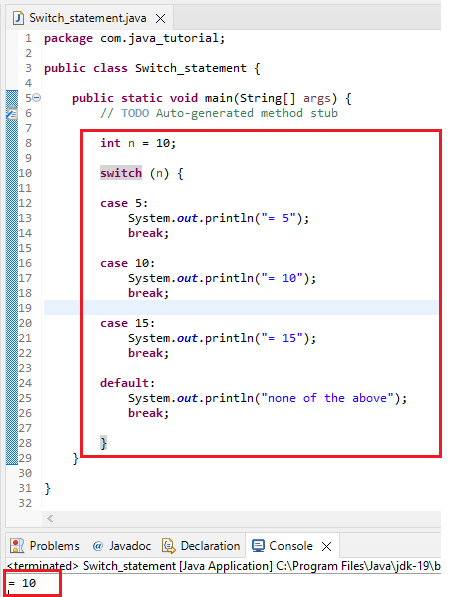
package com.java_tutorial;
public class Switch_statement {
public static void main(String[] args) {
// TODO Auto-generated method stub
int n = 10;
switch (n) {
case 5:
System.out.println("= 5");
break;
case 10:
System.out.println("= 10");
break;
case 15:
System.out.println("= 15");
break;
default:
System.out.println("none of the above");
break;
}
}
}
Example 2:
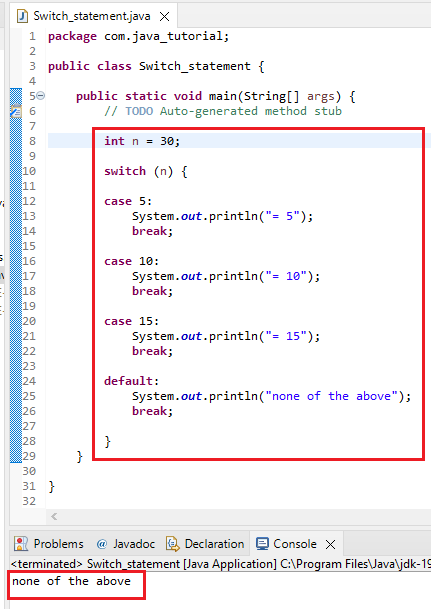
package com.java_tutorial;
public class Switch_statement {
public static void main(String[] args) {
// TODO Auto-generated method stub
int n = 30;
switch (n) {
case 5:
System.out.println("= 5");
break;
case 10:
System.out.println("= 10");
break;
case 15:
System.out.println("= 15");
break;
default:
System.out.println("none of the above");
break;
}
}
}
Comments (No)