Stack in Python:
We can use the PyCharm code editor for this example. If you do not know about it then follow this link- How to install PyCharm for Python and create a program in it. |
(1) A stack is a very useful data structure. It is simply a list with one restriction, Last In First Out (LIFO), meaning an element that comes in last goes out first when a value is read from a stack. Stack has two main operations called push and pop. When an element is added on the top of the stack it is called a push function and when an element is removed from the top of the stack, the operation is called a pop function. Other operations include peek and display. The Peek function displays the last element and the display function displays the full stack.
(2) Example of Implementation of Stack in Python:
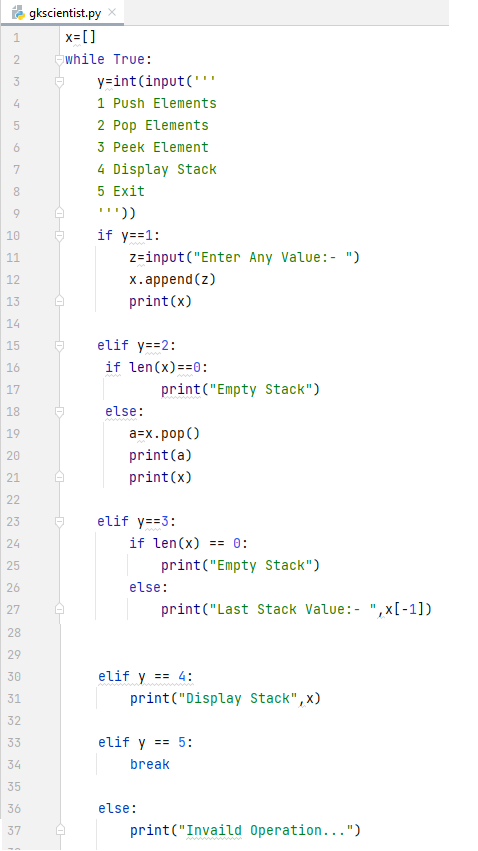
Now run the code, and you got the following output as shown below.
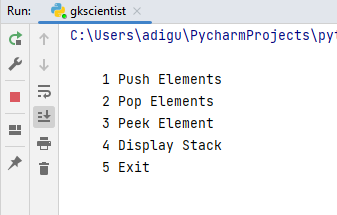
Now press 1 in the output window, in order to use the Push operation. After pressing 1, you got the message “enter any value”. You add any value of your choice. If you want to add another value then again click on 1 and you got the same message “enter any value”. You can add as many values as you want. The output is shown below.
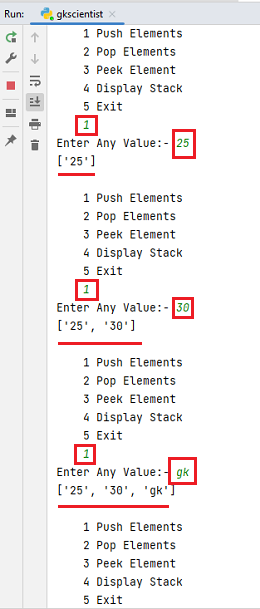
Now the list is created by using the push operation. Now press 2 in the output window, in order to use the Pop operation. After pressing 2, ‘gk’ will be dropped from the list as shown below.
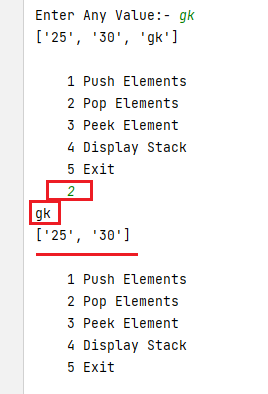
Now press 3 in the output window, in order to use the Peek operation. It displays the last element in the list as shown below.
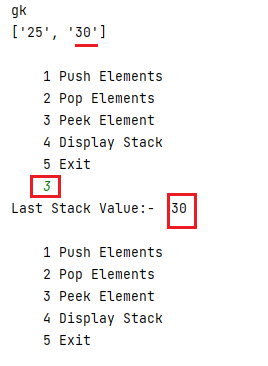
Now press 4 in the output window, in order to use the Display operation. This operation displays the full stack as shown below. You got only two values in the list because you have dropped the third value already by using the pop operation.
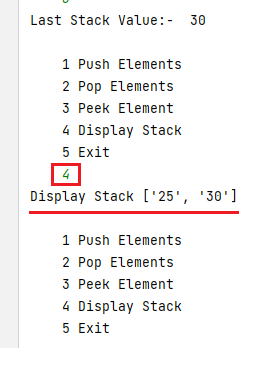
If you press 5, the process is getting finished.
Note: In the above example, we have performed a step-by-step operation. If you press 2 before 1, then the pop operation comes into play and you got the message “Empty Stack” as we set in the code as shown above because you did not create the list, so there will be no data that is why you got the output “Empty Stack”. The same is apply to all conditions.
Comments (No)