Java Nested Loops:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
Java Nested loop means a loop statement inside another loop statement. For example, a while loop can be nested inside another while loop or a for loop can be nested inside another for loop. In fact, the nested loops do not need to be the same loop type, that is, a for loop can be nested inside a while loop, and a while loop can be nested inside a for loop. Nested loops may be useful if we are performing multiple operations, each of which has its own count or sentinel value.
Code:
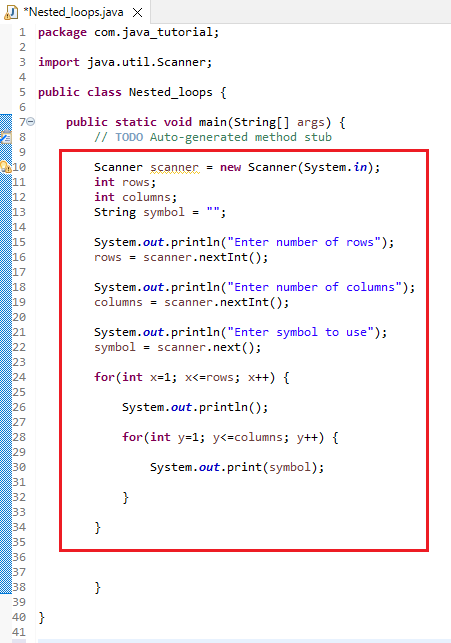
Output:
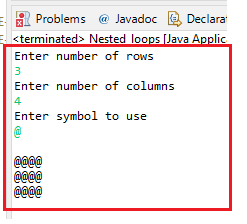
Java Nested Loops Source Code:
import java.util.Scanner;
public class Nested_loops {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner scanner = new Scanner(System.in);
int rows;
int columns;
String symbol = "";
System.out.println("Enter number of rows");
rows = scanner.nextInt();
System.out.println("Enter number of columns");
columns = scanner.nextInt();
System.out.println("Enter symbol to use");
symbol = scanner.next();
for(int x=1; x<=rows; x++) {
System.out.println();
for(int y=1; y<=columns; y++) {
System.out.print(symbol);
}
}
}
}
Comments (No)