Java StopWatch:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
In Java, we can create a StopWatch which contains three push buttons and one label. The push buttons are called Start, Stop and Reset and are used to start, stop and reset the StopWatch. The label displays the elapsed time. Although quite simple, this project shows the ease with which GUI interfaces can be created using Swing.
Sample Code for Java StopWatch:
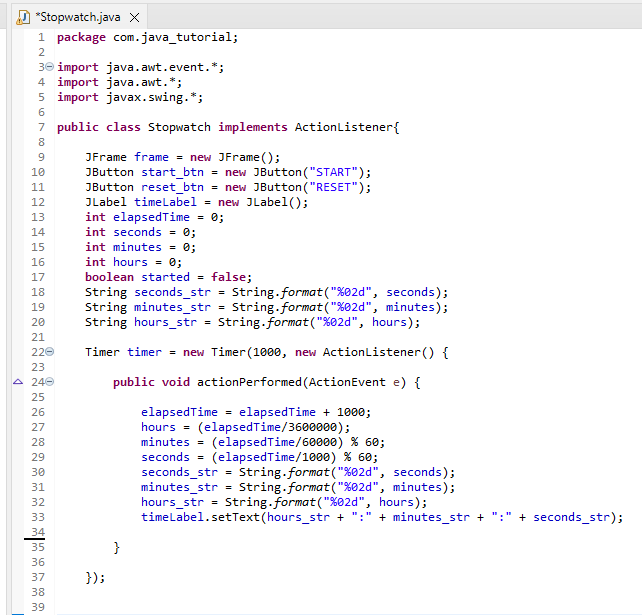
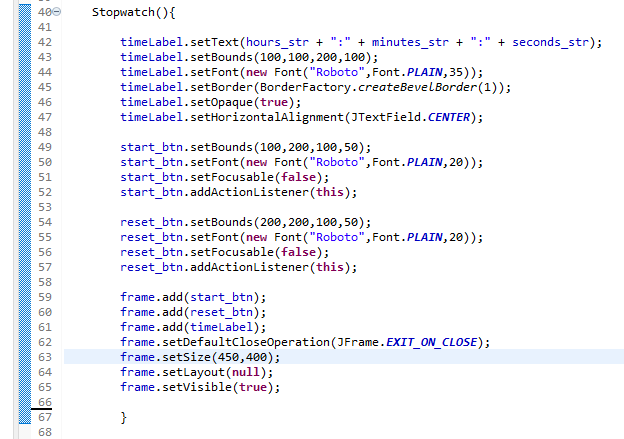
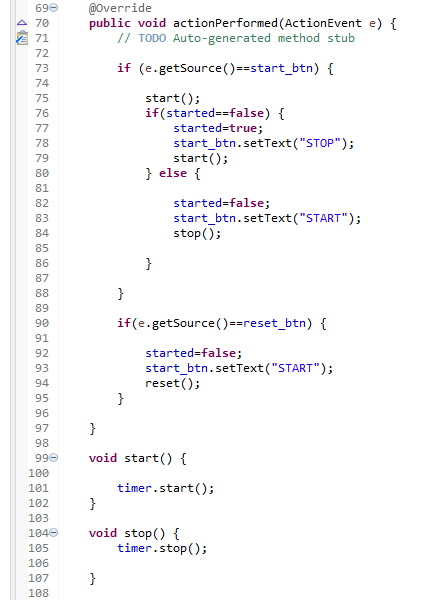
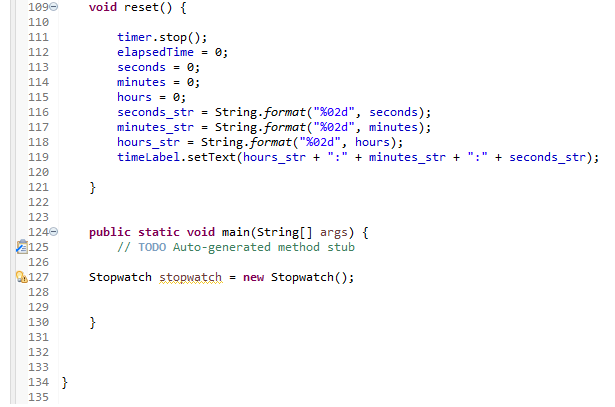
Output:
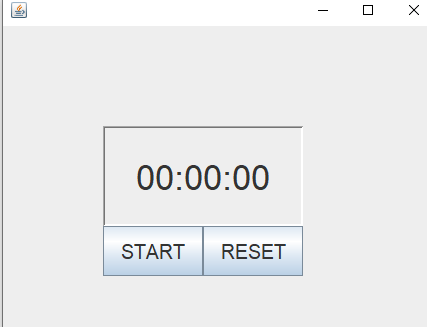
import java.awt.event.*;
import java.awt.*;
import javax.swing.*;
public class Stopwatch implements ActionListener{
JFrame frame = new JFrame();
JButton start_btn = new JButton("START");
JButton reset_btn = new JButton("RESET");
JLabel timeLabel = new JLabel();
int elapsedTime = 0;
int seconds = 0;
int minutes = 0;
int hours = 0;
boolean started = false;
String seconds_str = String.format("%02d", seconds);
String minutes_str = String.format("%02d", minutes);
String hours_str = String.format("%02d", hours);
Timer timer = new Timer(1000, new ActionListener() {
public void actionPerformed(ActionEvent e) {
elapsedTime = elapsedTime + 1000;
hours = (elapsedTime/3600000);
minutes = (elapsedTime/60000) % 60;
seconds = (elapsedTime/1000) % 60;
seconds_str = String.format("%02d", seconds);
minutes_str = String.format("%02d", minutes);
hours_str = String.format("%02d", hours);
timeLabel.setText(hours_str + ":" + minutes_str + ":" + seconds_str);
}
});
Stopwatch(){
timeLabel.setText(hours_str + ":" + minutes_str + ":" + seconds_str);
timeLabel.setBounds(100,100,200,100);
timeLabel.setFont(new Font("Roboto",Font.PLAIN,35));
timeLabel.setBorder(BorderFactory.createBevelBorder(1));
timeLabel.setOpaque(true);
timeLabel.setHorizontalAlignment(JTextField.CENTER);
start_btn.setBounds(100,200,100,50);
start_btn.setFont(new Font("Roboto",Font.PLAIN,20));
start_btn.setFocusable(false);
start_btn.addActionListener(this);
reset_btn.setBounds(200,200,100,50);
reset_btn.setFont(new Font("Roboto",Font.PLAIN,20));
reset_btn.setFocusable(false);
reset_btn.addActionListener(this);
frame.add(start_btn);
frame.add(reset_btn);
frame.add(timeLabel);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(450,400);
frame.setLayout(null);
frame.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
if (e.getSource()==start_btn) {
start();
if(started==false) {
started=true;
start_btn.setText("STOP");
start();
} else {
started=false;
start_btn.setText("START");
stop();
}
}
if(e.getSource()==reset_btn) {
started=false;
start_btn.setText("START");
reset();
}
}
void start() {
timer.start();
}
void stop() {
timer.stop();
}
void reset() {
timer.stop();
elapsedTime = 0;
seconds = 0;
minutes = 0;
hours = 0;
seconds_str = String.format("%02d", seconds);
minutes_str = String.format("%02d", minutes);
hours_str = String.format("%02d", hours);
timeLabel.setText(hours_str + ":" + minutes_str + ":" + seconds_str);
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Stopwatch stopwatch = new Stopwatch();
}
}
Comments (No)