We can use the PyCharm code editor for this example. If you do not know about it then follow this link- How to install PyCharm for Python and create a program in it. |
Python For Loop:
The for loops are used to execute repetitive tasks. A for loop will take a range of values as an argument and assign them one by one to a variable. The block of code will be executed once for each variable. The for loop is also used to work with objects that are iterable, such as sequences. So, with the help of this loop, you can iterate over each element that exists in a sequence of collections, get one element at a time and execute the code for that element.
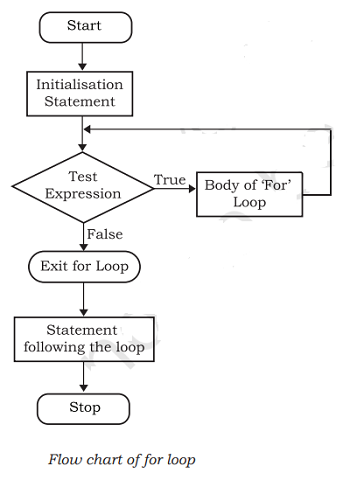
The Range() Function:
The range() is a built-in function in Python. It is used to create a list containing a sequence of integers from the given start value upto stop value (excluding stop value), with a difference of the given step value. To begin with, simply remember that function takes parameters to work on. In function range(), start, stop and step are parameters. The start and step parameters are optional. If the start value is not specified, by default the list starts from 0. If the step is also not specified, by default the value increases by 1 in each iteration. All parameters of the range() function must be integers. The step parameter can be a positive or a negative integer excluding zero.
Range() Function Example:
(1) range(5)
start = 0 condition < 5 increment 1 range function = 0, 1, 2, 3, 4 |
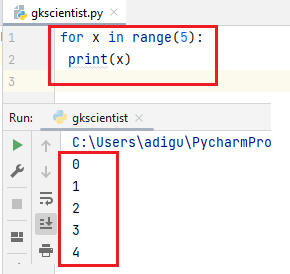
(2) range(1, 6)
start = 1 condition < 6 increment 1 range function = 1, 2, 3, 4, 5 |
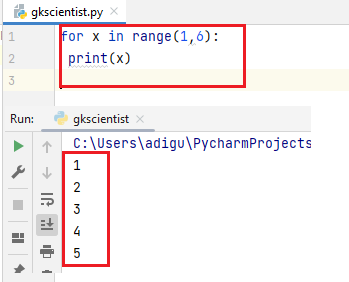
(3) range(1, 6, 2)
start = 1 condition < 6 increment 2 range function = 1, 3, 5 |
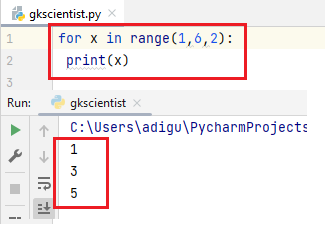
(4) Range Function Reverse case:
- range(5, 0, -1)
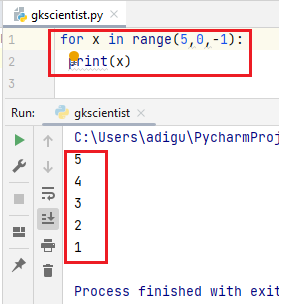
- range(8, 0, -2)
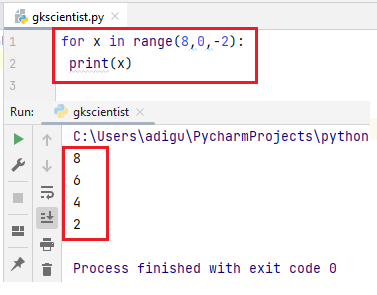
- range(20, 3, -4)
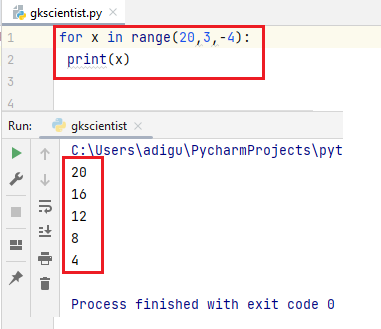
(5) Example- Create a table of 4 using for loop and range function.
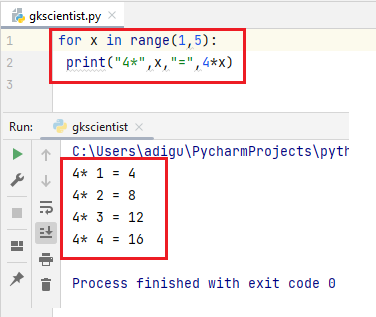
Comments (No)