Java TextField:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
Java TextField control creates a single-line text area. The TextField allows the user to enter and edit the text using cut, copy and paste keys, arrow keys, and mouse selections. It is an object of JTextField class which is a subclass of JTextComponent.
Some of the constructors defined by JTextField class are as follows-
- JTextField( )
- JTextField(int cols)
- JTextField(String string, int cols)
- JTextField(String string)
Where string is the initial string contained in the TextField and cols is the width of the TextField in terms of columns.
Sample Code for Java TextField:
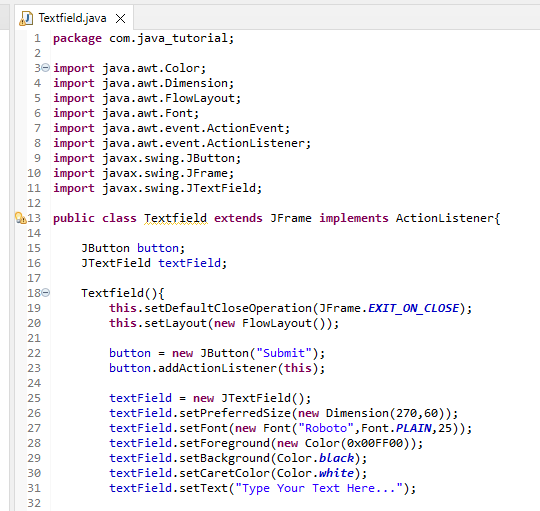
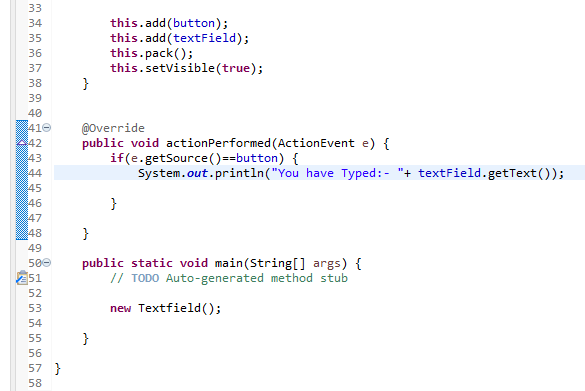
Output:
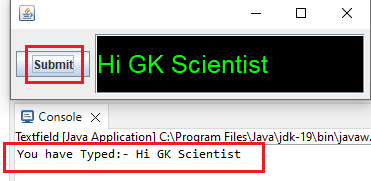
import java.awt.Color;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JTextField;
public class Textfield extends JFrame implements ActionListener{
JButton button;
JTextField textField;
Textfield(){
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setLayout(new FlowLayout());
button = new JButton("Submit");
button.addActionListener(this);
textField = new JTextField();
textField.setPreferredSize(new Dimension(270,60));
textField.setFont(new Font("Roboto",Font.PLAIN,25));
textField.setForeground(new Color(0x00FF00));
textField.setBackground(Color.black);
textField.setCaretColor(Color.white);
textField.setText("Type Your Text Here...");
this.add(button);
this.add(textField);
this.pack();
this.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if(e.getSource()==button) {
System.out.println("You have Typed:- "+ textField.getText());
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
new Textfield();
}
}
Comments (No)