Create ProgressBar in Java:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
A ProgressBar is a component that visually displays a value within an interval, and typically represents the progress of a certain task being performed such as software installation, etc. It can be of two types namely, Horizontal ProgressBar and Vertical ProgressBar. In Horizontal ProgressBar, the progress is indicated by filling the bar from left to right, and in Vertical ProgressBar, the progress is indicated by filling the bar from bottom to top.
Java ProgressBar Constructors:
Constructor | Description |
---|---|
JProgressBar( ) | Creates a new horizontal ProgressBar that displays a border but no progress string. The initial and minimum values are 0, and the maximum is 100. |
JProgressBar(int orient) | Creates a new ProgressBar with the specified orientation, which can be either JProgressBar.VERTICAL or JProgressBar.HORIZONTAL. |
JProgressBar(int min, int max) | Creates a new ProgressBar with the specified minimum value and maximum value. |
JProgressBar(int orient, int min, int max) | Creates a new ProgressBar with the specified orientation, minimum value and maximum value. |
Java ProgressBar Methods:
Method | Description |
---|---|
void setValue(int n) | Sets the ProgressBar current value. |
void setString(String s) | Sets the value of the progress string. |
void setOrientation(int newOrientation) | Sets the ProgressBar orientation to newOrientation, which must be JProgressBar.VERTICAL or JProgressBar.HORIZONTAL. |
void setMinimum(int n) | Sets the ProgressBar minimum value. |
void setMaximum(int n) | Sets the ProgressBar maximum value. |
Sample Code to Create ProgressBar in Java:
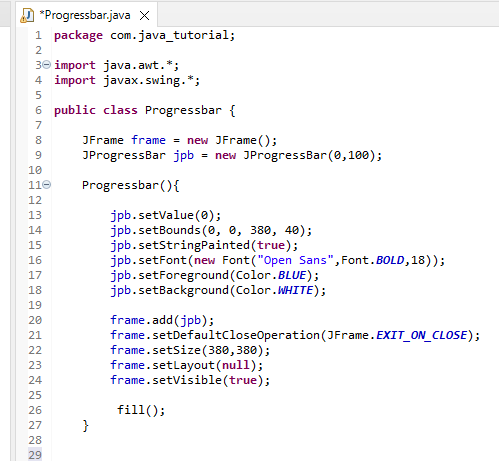
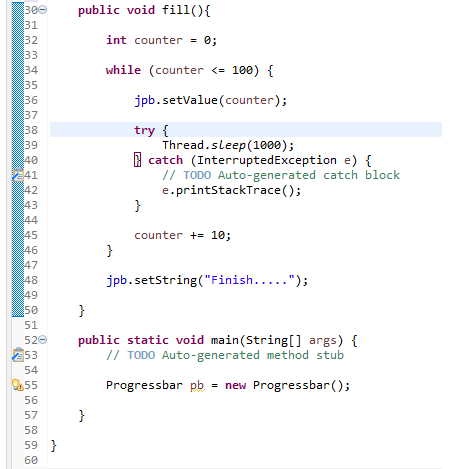
Output:
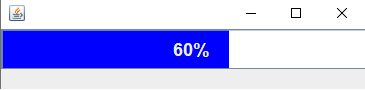
import java.awt.*;
import javax.swing.*;
public class Progressbar {
JFrame frame = new JFrame();
JProgressBar jpb = new JProgressBar(0,100);
Progressbar(){
jpb.setValue(0);
jpb.setBounds(0, 0, 380, 40);
jpb.setStringPainted(true);
jpb.setFont(new Font("Open Sans",Font.BOLD,18));
jpb.setForeground(Color.BLUE);
jpb.setBackground(Color.WHITE);
frame.add(jpb);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(380,100);
frame.setLayout(null);
frame.setVisible(true);
fill();
}
public void fill(){
int counter = 0;
while (counter <= 100) {
jpb.setValue(counter);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
counter += 10;
}
jpb.setString("Finish.....");
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Progressbar pb = new Progressbar();
}
}
Comments (No)