Java Random Class:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
Java’s Random class generates sequences of numbers that, by the most stringent technical standards, are “almost random”. To use the correct terminology, the Random class creates a pseudo-random sequence of numbers.
Constructor for Java Random Class:
Constructor | Description |
---|---|
Random() | Creates a new random number generator. |
Random(seed:long | Creates a new random number generator using a single long seed. |
Java Random Class Methods:
Method | Description |
---|---|
nextInt() | Returns the random integer value. |
nextInt(n) | Returns the random integer value from the range 0 to n. |
nextLong() | Returns a random long value. |
nextDouble() | Returns a random double value. |
nextFloat() | Returns a random float value. |
nextBoolean() | Returns a random boolean value. |
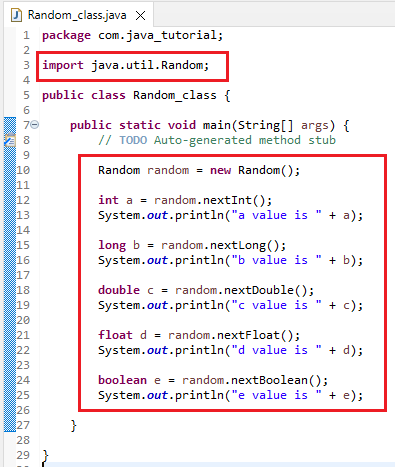
Output 1:
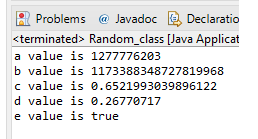
Output 2:
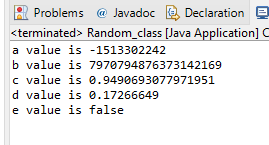
package com.java_tutorial;
import java.util.Random;
public class Random_class {
public static void main(String[] args) {
// TODO Auto-generated method stub
Random random = new Random();
int a = random.nextInt();
System.out.println("a value is " + a);
long b = random.nextLong();
System.out.println("b value is " + b);
double c = random.nextDouble();
System.out.println("c value is " + c);
float d = random.nextFloat();
System.out.println("d value is " + d);
boolean e = random.nextBoolean();
System.out.println("e value is " + e);
}
}
Comments (No)