Scanner Class in Java:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
Scanner Class in Java allows us to input or read the primitive data types. It is a class in java.util package used for obtaining the input of primitive types like int, double, and strings. It is one of the easiest ways to receive input in a Java program. The java.util.Scanner is a simple text scanner that can parse primitive types and strings using regular expressions. A scanner breaks its input into tokens using a delimiter pattern, which by default matches whitespace.
Example 1: Using one String and one Integer in the code as shown below.
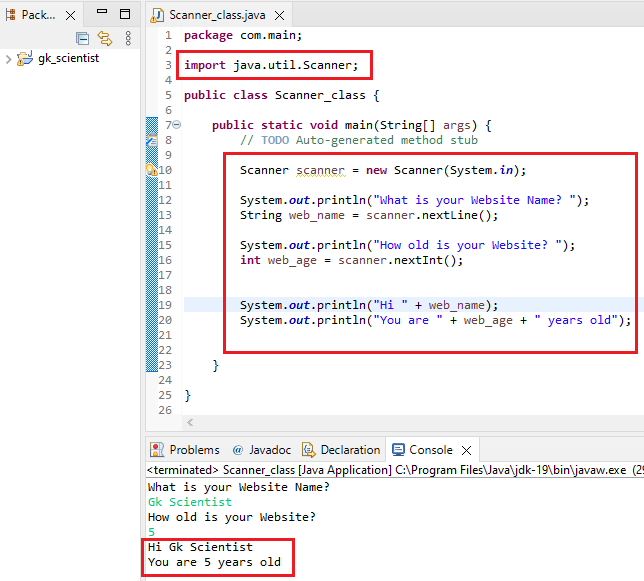
package com.main;
import java.util.Scanner;
public class Scanner_class {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner scanner = new Scanner(System.in);
System.out.println("What is your Website Name? ");
String web_name = scanner.nextLine();
System.out.println("How old is your Website? ");
int web_age = scanner.nextInt();
System.out.println("Hi " + web_name);
System.out.println("You are " + web_age + " years old");
}
}
Example 2: Using two String and one Integer in the code as shown below.
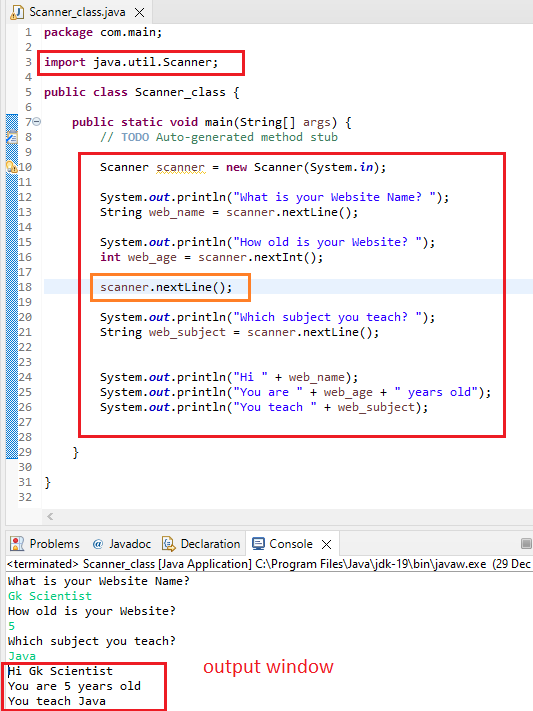
package com.main;
import java.util.Scanner;
public class Scanner_class {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner scanner = new Scanner(System.in);
System.out.println("What is your Website Name? ");
String web_name = scanner.nextLine();
System.out.println("How old is your Website? ");
int web_age = scanner.nextInt();
scanner.nextLine();
System.out.println("Which subject you teach? ");
String web_subject = scanner.nextLine();
System.out.println("Hi " + web_name);
System.out.println("You are " + web_age + " years old");
System.out.println("You teach " + web_subject);
}
}
Comments (No)