Java FileReader Class:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
The FileReader class provides basic methods for reading data from a character stream that originates from a file. It provides methods that let you read data one character at a time. Usually, you don’t work with this class directly. Instead, you create a FileReader object to connect your program to a file and then pass that object to the constructor of the BufferedReader class, which provides more efficient access to the file. (This class extends the abstract class Reader, which is the base class for a variety of classes that can read character data from a stream).
Java FileReader Class Constructors:
Constructor | Description |
---|---|
FileReader (String file) | It gets the filename as a string. It opens the specified file in read-only mode. If a file does not exist, the FileNotFoundException is thrown. |
FileReader (File file) | It gets the filename from the file instance. It opens the specified in read-only mode. If the file does not exist, the FileNotFoundException is thrown. |
Java FileReader Class Methods:
Method | Description |
---|---|
int read () | It is used to return an ASCII character. At the conclusion of the file, it returns -1. |
void close () | It is used to close the FileReader class. |
In the below example, we copy the ASCII image art from the ASCII Art Archive website and paste it into the notepad. Now we save the notepad file. Copy that file and paste it into the java project folder as shown.
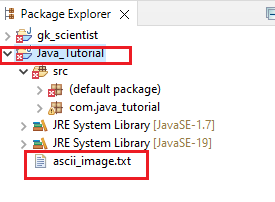
After that write the below code to read the notepad file i.e. ascii_image.txt.
Java FileReader Class Code Unit:
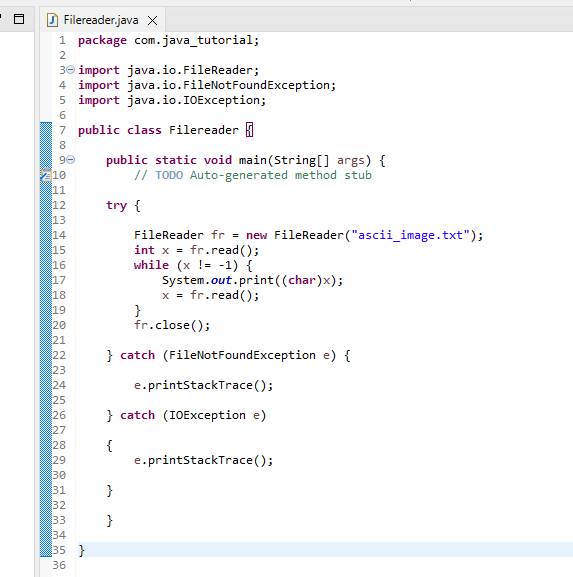
Java FileReader Class Output:
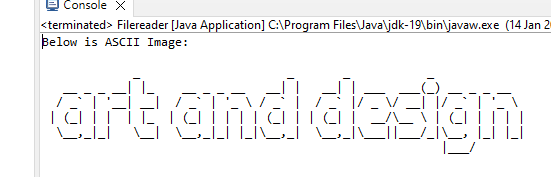
import java.io.FileReader;
import java.io.FileNotFoundException;
import java.io.IOException;
public class Filereader {
public static void main(String[] args) {
// TODO Auto-generated method stub
try {
FileReader fr = new FileReader("ascii_image.txt");
int x = fr.read();
while (x != -1) {
System.out.print((char)x);
x = fr.read();
}
fr.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e)
{
e.printStackTrace();
}
}
}
Comments (No)