Java while Loop:
We can use the Eclipse IDE for this example. If you do not know about it then follow this link- How to Install Eclipse For Java and create a program in it. |
A while loop keeps executing the statement in its body (which may be a compound statement, with a number of single statements inside curly braces) while a particular condition evaluates to true. Note that if the condition is not true, the body of the loop is not even executed once.
Syntax of Java while Loop:
while (condition) { statements; } |
The do-while loop is just like a while loop, except that the test condition is evaluated at the end of the loop, not at the beginning.
Syntax of Java do-while Loop:
do { statements; } while (condition); |
Example 1:
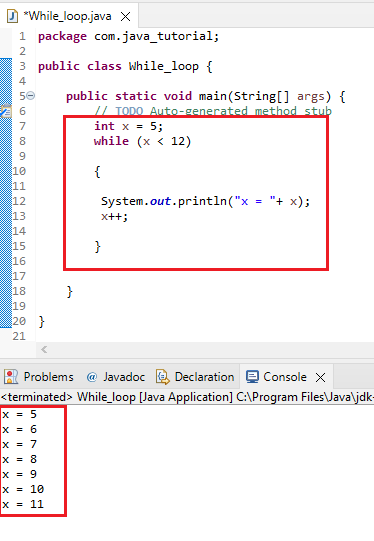
public class While_loop {
public static void main(String[] args) {
// TODO Auto-generated method stub
int x = 5;
while (x < 12)
{
System.out.println("x = "+ x);
x++;
}
}
}
Example 2:
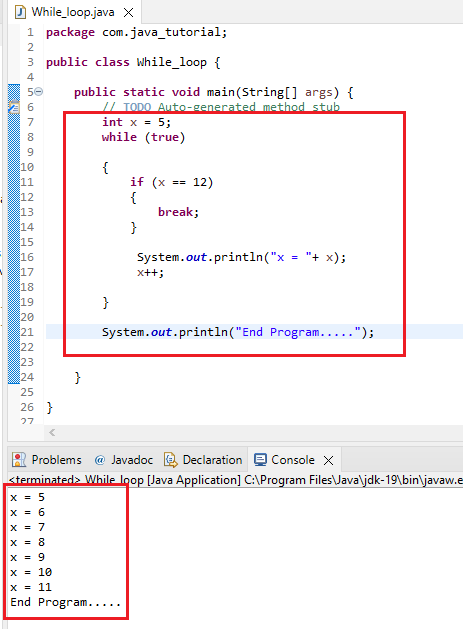
public class While_loop {
public static void main(String[] args) {
// TODO Auto-generated method stub
int x = 5;
while (true)
{
if (x == 12)
{
break;
}
System.out.println("x = "+ x);
x++;
}
System.out.println("End Program.....");
}
}
Example 3:
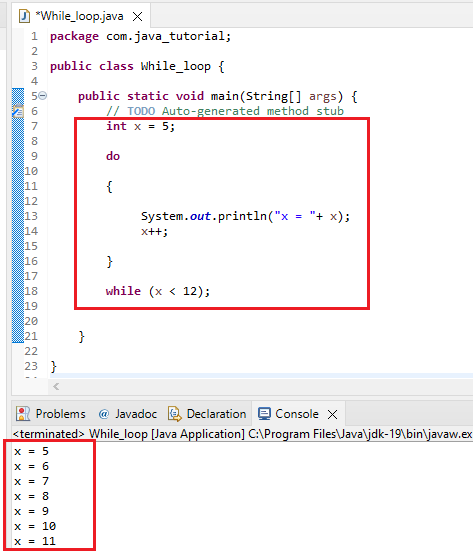
package com.java_tutorial;
public class While_loop {
public static void main(String[] args) {
// TODO Auto-generated method stub
int x = 5;
do
{
System.out.println("x = "+ x);
x++;
}
while (x < 12);
}
}
Example 4:
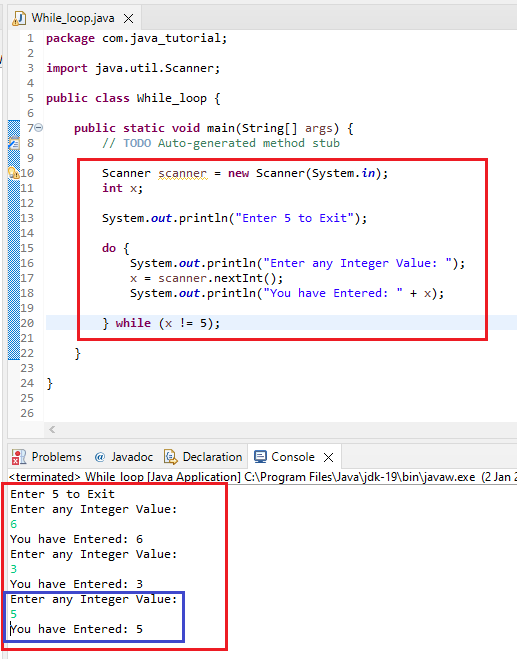
import java.util.Scanner;
public class While_loop {
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner scanner = new Scanner(System.in);
int x;
System.out.println("Enter 5 to Exit");
do {
System.out.println("Enter any Integer Value: ");
x = scanner.nextInt();
System.out.println("You have Entered: " + x);
} while (x != 5);
}
}
Note: The break statement is to be used only in loops and switch statements. When this statement is executed, the control is taken out of the loop. The loop becomes dead.
Comments (No)